PROJECT: StardyTogether
StardyTogether is a desktop contacts and venues manager application specially for NUS students to help them find free time and rooms to study together with their friends.
Most of the user interactions are via CLI, while there exists a GUI created with JavaFX. It is written in Java and has about 10kLoC.
The source code is based on the AddressBook-Level4 project created by SE-EDU initiative.
Code contributed: [Functional code]
Feature Contributions
-
Major enhancement: Timetable Attribute and Union Command
-
What it does: Allows students to import their NUSMods timetables into
-
Justification: This feature makes the adoption and use of StardyTogether much more convenient as students can now remove the hassle of manually entering their timetables and use StardyTogether along with their existing tools.
The union of the timetable also allows students to easily plan study sessions today during their free breaks. -
Credits: Timetable importing features obtains data from NUSMods API (http://api.nusmods.com)
-
-
Minor enhancement: Birthday Attribute and Command
-
What it does: Shows a list of birthdays and notification of birthdays occurring today
-
Justification: This allows students to fulfill their social obligations with their friends by reminding them of their friends' birthdays at the start of the app. Students can also open a list to plan for birthdays ahead of time.
-
-
Other contributions:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Viewing a collated birthday list/notification: birthdays
(since v1.3)
To get a list that contains all the birthdays of all your friends (ordered by date)
Or to know whose birthday is it today, you can enter the following commands
Format: birthdays [ADDITIONAL_PARAMETER]
The following parameters can be inputted into the [ADDITIONAL_PARAMETER] field
Examples:
-
birthdays
Displays a list of all your friends' birthdays
-
birthdays today
Displays a window with the birthdays occuring today
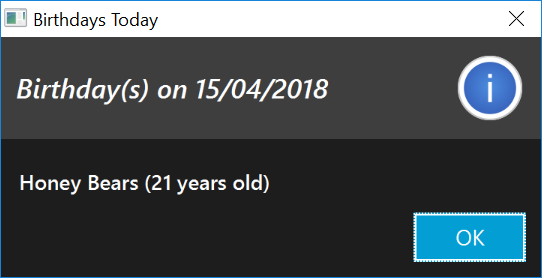
Viewing common time slots in timetable: union
(since v1.5rc)
To know your free slots together with your friends, you can use union
to display the common free time of multiple friends in ST. (Minimum: 2)
Format: union ODD/EVEN INDEX INDEX [INDEX]…
ODD/EVEN is case-insensitive |
Only indexes of the current filtered list are valid |
Examples:
-
union Odd 1 2 3
Displays the combined odd timetable for friends at Index 1, 2 and 3.
-
union Even 1 2
Displays the combined even timetable for friends at Index 1 and 2.
Modules Tag (coming in v2.0)
Each friend will be able to have their own list of modules they have taken.
To find out which friends have not taken a module (so as to plan with them to take), you can enter the command module
followed by the module code
Format: module MODULE_CODE
Module code follows the traditional case-sensitive naming conventions used by NUS (Example: CS2103T or CS2101) |
Examples:
-
module CS2103
Displays the list of friends who have not taken the module CS2103
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Birthdays feature
Current Implementation
Birthdays
Command uses the existing Events
system and sends an event according to the parameters provided.
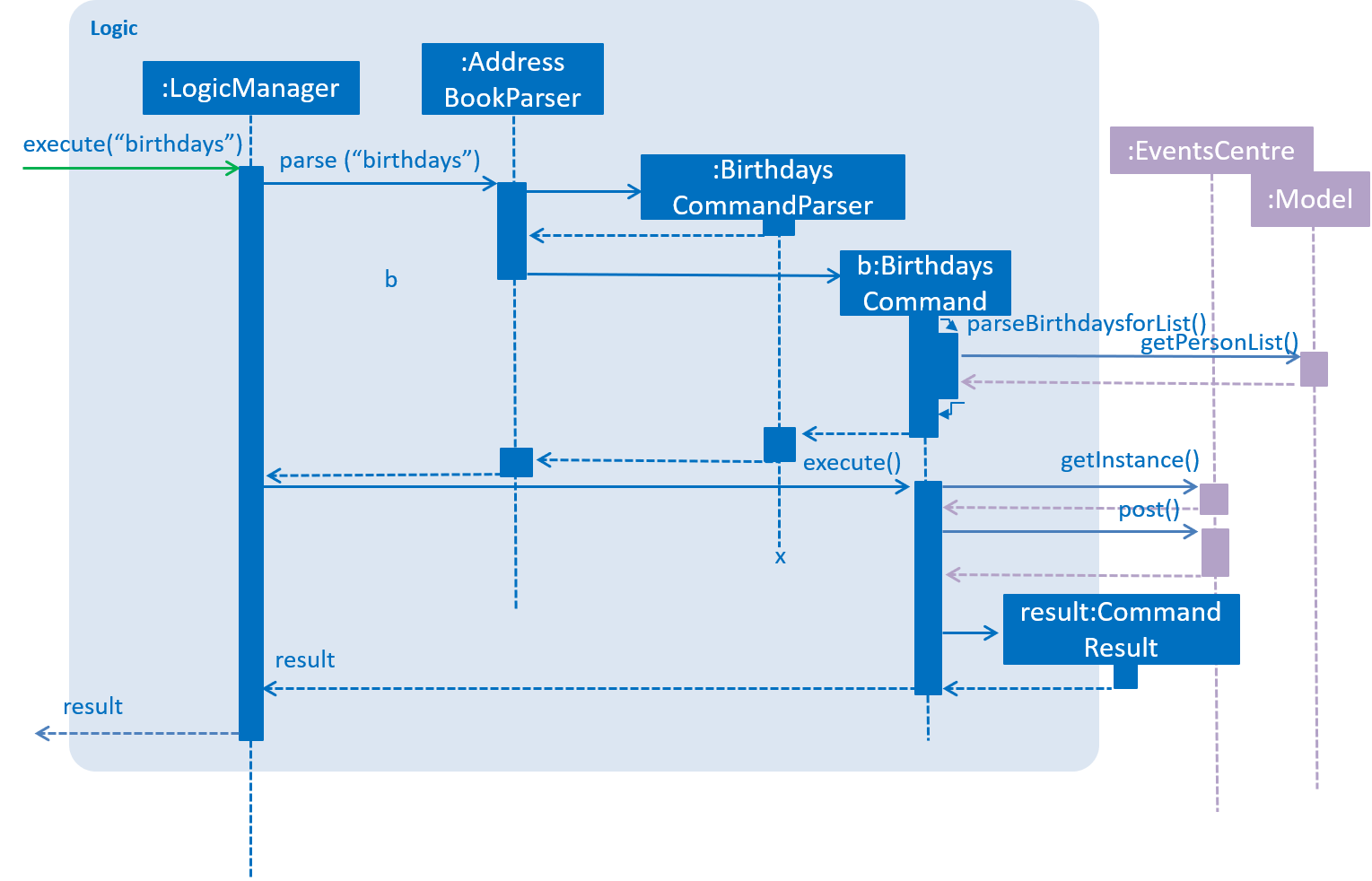
The BirthdayList
UI component will then receive the event and handle the display of the data
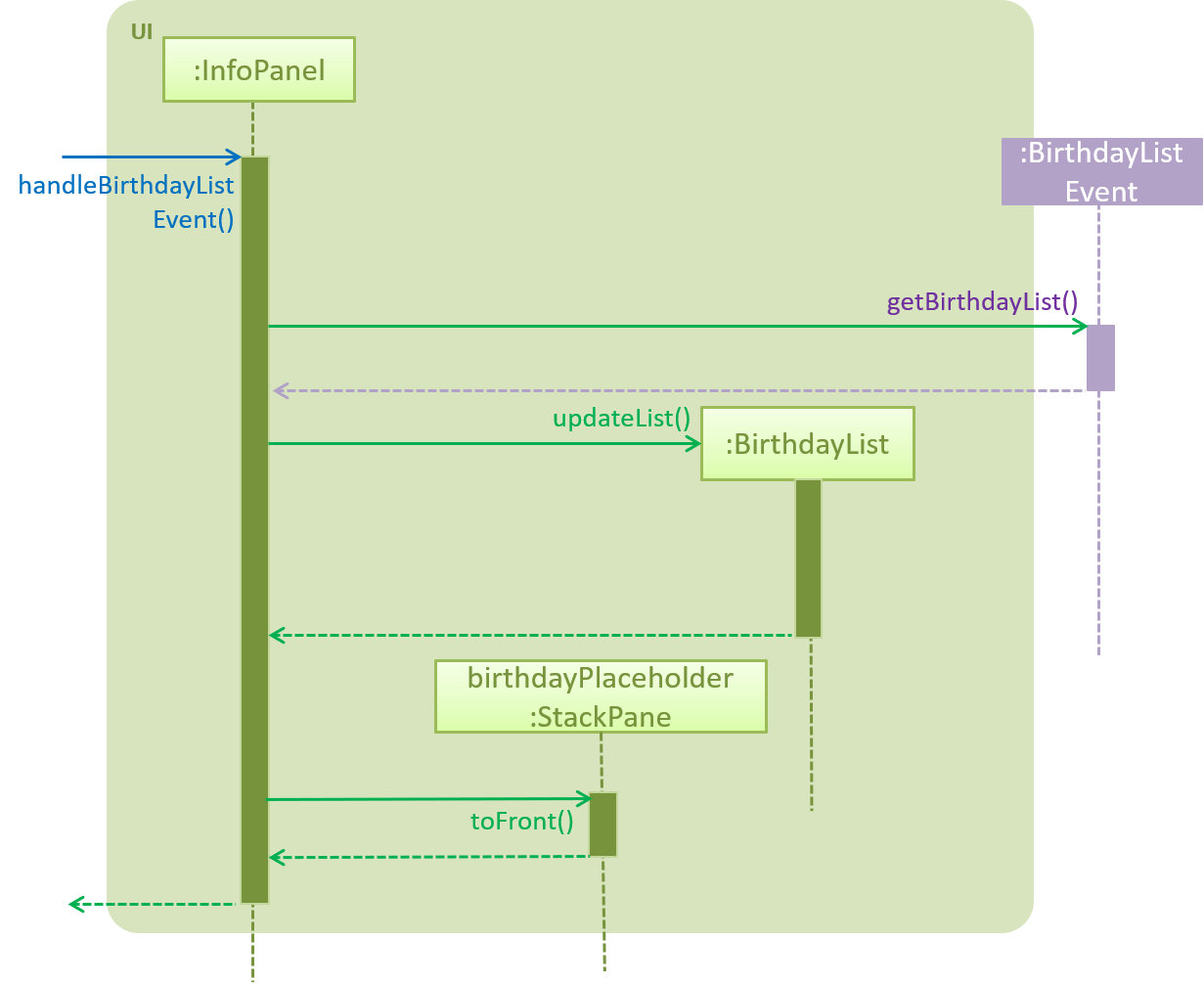
For "birthdays today" notification, the app will create an alert dialog instead.
@Subscribe
private void handleBirthdayNotificationEvent(BirthdayNotificationEvent event) {
DateTimeFormatter dtf = DateTimeFormatter.ofPattern("dd/MM/yyyy");
logger.info(LogsCenter.getEventHandlingLogMessage(event));
Alert alert = new Alert(Alert.AlertType.INFORMATION);
// ... setting up of Alert ...
alert.showAndWait();
}
Design Considerations
Aspect: How the BirthdayList
UI component obtains and parses its data
-
Alternative 1: Let UI component handle the parsing of UniquePersonList obtained from Event
-
Pros: Isolated and independent within
BirthdayList
UI component. Less overhead. -
Cons: Not intuitive to new developers as parsing of data is not expected in UI.
-
-
Alternative 2 (current choice): Let
Birthdays
do the parsing of UniquePersonList obtained from Model-
Pros: More modularity.
-
Cons: Not apparent in usage by User. Functionality remains the same but Birthdays command becomes more cluttered.
-
Aspect: How User can open Birthday List
-
Alternative 1: Manual command "birthdays" or "birthdays today"
-
Pros: User can control when to view the birthdays.
-
Cons: Not very user-friendly. Additional parameter cannot be shortened.
-
-
Alternative 2 (current choice): Notification at the start of app if a birthday is occurring today
-
Pros: User can be reminded immediately and need not type the command.
-
Cons: Currently, StardyTogether does not have settings to switch on/off the feature. User may find it irritating.
-
Aspect: How User inputs the Birthday parameter in Person
class
-
Alternative 1 (Current choice): Fixed format as DDMMYYYY
-
Pros: Less room for errors.
-
Cons: User may not like the DDMMYYYY format.
-
-
Alternative 2: Use Natural Language Processing
-
Pros: Users can enter their birthday in their preferred format.
-
Cons: External API will be used. May introduce unforeseen bugs.
-
Timetable feature
Current Implementation
When adding a Person
using the "Add" Command, users can enter their NUSMods shortened link into the "tt/" field.
NUSMods URLs currently come in the format of …/timetable/SEM_NUM/share?MODULE_CODE=LESSON_CODE
Using TimetableParserUtil:parseShortUrl
, we obtain the full url from the shortened link.
Then, we parse the information accordingly and obtain lesson data from [NUSMods API] to represent them in Lesson
The information is then sorted and added as a list of Lesson
taken by the user to the Timetable.
try {
// Grab lesson info from API and store as a map
URL url = new URL(link);
@SuppressWarnings("unchecked")
Map<String, Object> mappedJson = mapper.readValue(url, HashMap.class);
@SuppressWarnings("unchecked")
ArrayList<HashMap<String, String>> lessonInfo = (ArrayList<HashMap<String, String>>)
mappedJson.get("Timetable");
// Parse the information from API and creates an Arraylist of all possible lessons
ArrayList<Lesson> lessons = new ArrayList<>();
for (HashMap<String, String> lesson : lessonInfo) {
Lesson lessonToAdd = new Lesson(moduleCode, lesson.get("ClassNo"), lesson.get("LessonType"),
lesson.get("WeekText"), lesson.get("DayText"), lesson.get("StartTime"), lesson.get("EndTime"));
lessons.add(lessonToAdd);
}
return lessons;
} catch (IOException exception) {
throw new ParseException("Cannot retrieve module information");
}
The main contents of the timetable is stored as TimetableData
and is accessed through Timetable
.
TimetableData
consists of 2 TimetableWeek
, which each consist of 5 TimetableDay
, which each consist of 24
TimetableSlot
(following the 24h clock)
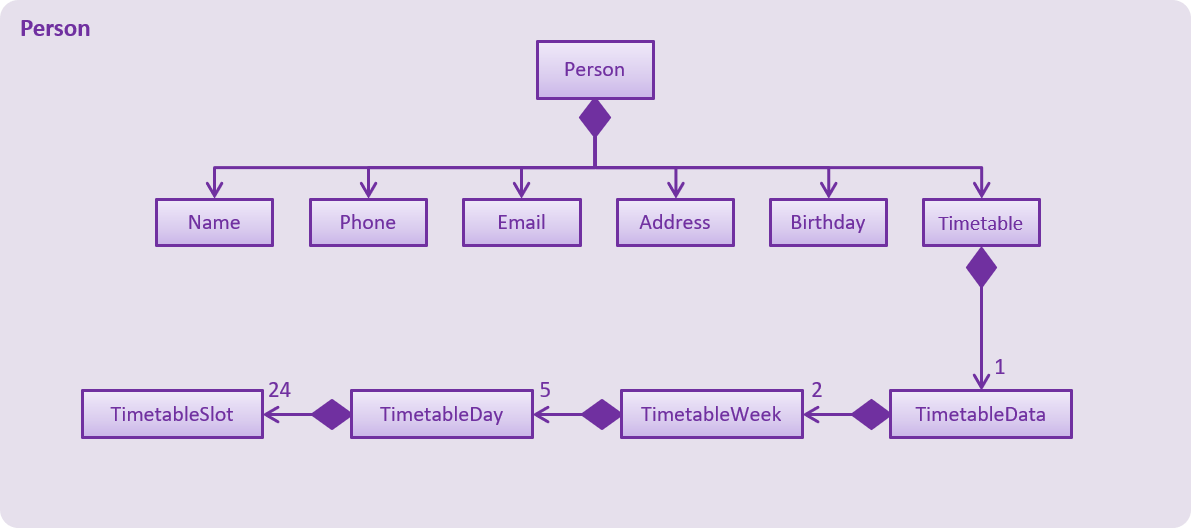
In the event the url provided is invalid or empty, a empty Timetable
will be created.
Do take note that there are dummy urls for the purpose of testing. While normal users should not be able to know of their existence,
entering a dummy link will result in a preset timetable being built.
When the user uses the TimetableUnionCommand
, the indexes selected will be parsed and a union of the timetables selected will be created.
public static ArrayList<String> unionTimetableDay(ArrayList<TimetableDay> timetables) {
ArrayList<String> commonTimetable = new ArrayList<>();
boolean checker;
for (int i = 8; i < 22; i++) {
checker = false;
for (TimetableDay timetable : timetables) {
TimetableSlot t = timetable.timetableSlots[i];
if (!t.toString().equals(EMPTY_SLOT_STRING)) {
checker = true;
break;
}
}
if (checker) {
commonTimetable.add(TITLE_OCCUPIED);
} else {
commonTimetable.add(EMPTY_SLOT_STRING);
}
}
return commonTimetable;
}
Afterwards, it will raise the TimeTableEvent
which will be caught and handled by the InfoPanel
.
The InfoPanel
will swap between UserDetailsPanel
, BirthdayList
, VenueTable
and TimetableUnionPanel
so that the UI would not be too cluttered.
Design Considerations
Aspect: The use of NUSMods Shortened URLs
-
Alternative 1 (current choice): Use NUSMods shortened urls to 'import' the user’s timetable over to StardyTogether
-
Pros: User-friendly if user already uses NUSMods and knows how to get the shortened link
-
Cons: Not helpful to a user who does not use NUSMods. If NUSMods API changes, StardyTogether needs to be updated
-
-
Alternative 2: Allow the use of more universal formats such as .ics files
-
Pros: More flexibility for the user
-
Cons: Hard to implement and parse the input
-
Aspect: Behaviour of the app when data from API is not retrieved successfully
-
Alternative 1 (current choice): A empty timetable is created for them.
-
Pros: Prevents unexpected errors
-
Cons: Not very intuitive unless user sees the thrown exception
-
-
Alternative 2: Prevent the adding of a Person without a valid timetable
-
Pros: Warns the user that the timetable is not inputted properly
-
Cons: Not very user-friendly if user just does not have a valid timetable
-
Aspect: Adding of lessons to Timetable
-
Alternative 1 (current choice): Users do the adding on NUSMods and re-import the timetable link
-
Pros: No need to implement a separate function to add lessons and a separate
Module
class -
Cons: May be troublesome for the user
-
-
Alternative 2: Implement a function to add lessons and
Module
class-
Pros: User need not to manually edit the timetable parameter
-
Cons: Hard to implement. Lessons and modules will not have any usage outside
Timetable
-
Aspect: Testing of Timetable
-
Alternative 1 (current choice): Dummy links (which will never be generated by NUSMods) are used, Timetable will parse those differently
-
Pros: Allows for easy creation of dummy timetables
-
Cons: Although unlikely, user may be able to enter the dummy link as his own timetable (unintended behaviour)
-
-
Alternative 2: Changing value to be non-final, settable with a method
-
Pros: Easy to implement
-
Cons: Violates coding conventions, allows possible unauthorized access to Timetable
-
Aspect: Displaying of Timetable in UI
-
Alternative 1 (Current choice): Change between the different panels
-
Pros: UI would not be too cluttered.
-
Cons: User cannot simultaneously use the different panels.
-
-
Alternative 2: Have a dedicated spot in the UI for
TimeTablePanel
-
Pros: Easy to refer for users.
-
Cons: UI would be confusing and cluttered.
-
Aspect: Size of Timetable size
-
Alternative 1 (Current choice): Automatically resize according to the size of the Application
-
Pros: Size is adaptable to the size of the Application.
-
Cons: Variable size may make it confusing for users.
-
-
Alternative 2: Fixed Size
-
Pros: Easy and predictable size and location of timings.
-
Cons: Since display may different from computer to computer, it would be inflexible to use a one size fit all approach.
-
Aspect: Color of Modules in Timetable
-
Alternative 1 (Current choice): Automatically randomized based on the
hashcode()
of the module name-
Pros: Colors are fixed and more or less randomized.
-
Cons: Colors may be same for different modules in the same timetable and Colors are not customizable.
-
-
Alternative 2: Pre-defined colors for the different modules
-
Pros: No overlap in color and different color for each module
-
Cons: Since there are many different modules in NUS, it would be very time-consuming and almost impossible to be implemented.
-
-
Alternative 3: User customize colors
-
Pros: Customized Application for users.
-
Cons: Implementation of this system would be complex and time-consuming, it would be implemented in later versions. Current implementation is the best in terms of variability and ease of implementation.
-